Entries tagged "PHP"
Using PHP to Dynamically Hide Content after an Expiration Date
If you need to pull something offline by a specific date, what happens when no one's around to remove the content? People get sick or maybe a deadline was overlooked and you're out of the office. Well you could just suck it up, go into work, and remove the registration form from the website. Or you could write a little PHP code to disable the form (or any other information) for you. [Continue reading]
Maintaining Line Breaks When Copying Code from PHPFreaks.com
While helping people on PHPFreaks.com, I tend to have issues copying code from their forum and pasting it into Dreamweaver. For some reason all the line breaks are removed from the code. As much as I love manually adding dozens of line breaks back into the code, there has to be a quicker solution. [Continue reading]
How to Turn Off Curly Quotes in WordPress so They Don’t Break Your PHP Tutorials
Have you ever copied PHP code from a website tutorial, but no matter what you did you couldn't get it to work? Or maybe you're posting code on a WordPress blog and can't figure out why people are saying the code doesn't work? Well you're not alone. You may have been bitten by the curly quote bug in WordPress. [Continue reading]
Setting up a Makeshift Test Environment for Experimenting with PHP Code
There are times I need to experiment with new code or re-familiarizing myself with code already in use. But there are times when other project code (sending out e-mails, updating databases, etc.) gets in the way of the tests. The code could be commented out to prevent it from executing, but that may be more work than it's worth. Plus I may forget to uncomment something when the tests are over. Instead, it can be easier to create a new file and focus on the code at hand. [Continue reading]
Security Issues with “You Are Now Leaving Our Website” Pages
If you've visited a government website, there's a good chance that you've seen the "You are now leaving our website" message. The message, as you have probably guessed, is displayed when a visitor clicks a link leading to an external website. Now I don't plan to discuss the validity of this technique but the potential security risk if utilized incorrectly. [Continue reading]
Developing a Simple Website Template with PHP
When developing websites it's always a good idea to look for ways to make the final product easier to maintain. For websites which contain more than a couple of pages, it can be a real time saver if you build the website utilizing a template.
To build a template you need to identify the components which stay the same from page to page throughout your website. For example, there probably is a page header which contains your organization's logo and/or name. What about a main navigation area that links to the main sections of your website? How about a page footer?
Background
Before getting our hands dirty, let's talk a little about what our example website looks like behind the scenes. Not the code, but the directory structure. Websites typically consist of many files and folders. In Figure 1 below, you can see the example website is made up of four folders and at least one PHP file.
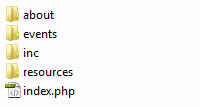
Figure 1. Directory Structure for the Example Website
Building the Template
So let's say the index.php file contains the following code and we want to create a template component for the main navigation:
<body>
<div id="page_header">Page Header</div>
<div id="main_navigation">
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about/">About Us</a></li>
<li><a href="/resources/">Resources</a></li>
<li><a href="/events/">Events</a></li>
</ul>
</div>
<div id="main_content">
<h1>Main Content</h1>
…
First we need to highlight and cut the main navigation code out of index.php. Then we'll paste the code into a new file called main_navigation.html which will be saved in the "inc" folder. We can now import the code back into index.php using the require() function provided by PHP.
<body>
<div id="page_header">Page Header</div>
<?php
//IMPORT THE MAIN NAVIGATION
require($_SERVER['DOCUMENT_ROOT'] . '/inc/main_navigation.html');
?>
<div id="main_content">
<h1>Main Content</h1>
…
Now you just need to repeat the process for any other template components you may have. In the example code above, we'll also want to replace the page header code:
<body>
<?php
//IMPORT THE PAGE HEADER
require($_SERVER['DOCUMENT_ROOT'] . '/inc/page_header.html');
//IMPORT THE MAIN NAVIGATION
require($_SERVER['DOCUMENT_ROOT'] . '/inc/main_navigation.html');
?>
<div id="main_content">
<h1>Main Content</h1>
…
Once all the template files are in place, you can start creating new pages based on the template. You just need to duplicate one of the files already using the template and customize the page as necessary.
Conclusion
The benefit for using templates is when you need to change something; you only need to update one file. For example, to include a contact us link in the main navigation for all of your pages, you only need to update the "main_navigation.html" file and you're done.
In addition to reducing the maintenance time require for a website, templates make it easier to add things like the Google Analytics tracking code. Or maybe you want to add a search engine to all of your pages. Templates give you the hook needed to easily incorporate content throughout your website.
Related Resources
- PHP Manual: require() – describes the require() and include() functions in more detail.
- PHP Manual: $_SERVER – help documentation for $_SERVER['DOCUMENT_ROOT'].
Future Proofing Your Google Analytics Code for Tracking PDFs
A few years back I started using Google Analytics to get a better idea of what people are viewing on our websites. To be prepared for future revisions of the tracking code, I thought all I needed to do was store the code in a file called "GoogleAnalytics.html" and import it into the pages we wanted to track. Then as Google releases new versions of the tracking code, I would only need to update the GoogleAnalytic.html file for each website.
Unfortunately, you can't add the tracking code to every file on the website. To track how many visitors open a PDF for example, you have to add extra code to the page that links to the PDF. Let's say you wanted to link to the Spring 2010 newsletter. The tracking code would likely be added to the anchor tag using the "onclick" attribute as follows:
The problem was that I didn't think about future proofing this part of the tracking code. So when the time arrived to update the analytics code, I needed to replace more than 230 onclick references throughout one website.
So instead of hard-coding the onclick attribute like before, I wanted to create a PHP function that writes the tracking code for me. In addition to being able to create tracking code for PDFs, Word documents, PowerPoint files, etc., the function should be able to handle links to other websites.
Building the Function
First I defined a PHP function called "addTrackingCode". The function accepts one argument ($link) which is used to send the link to track.
function addTrackingCode($link) {
}
Inside the function, I want optimize the link for Google Analytics.
function addTrackingCode($link) {
    //IF THE LINK LEADS TO MY WEBSITE (http://www.mywebsite.com/newsletter/2010spr.pdf), REMOVE THE "http://" AND THE DOMAIN NAME
     if(substr($link, 0, 24)=='http://www.mywebsite.com') {
         $link = substr($link, 24);
         Â
    //ELSE…IF THE LINK STARTS WITH "http", IT'S AN EXTERNAL LINK
    } elseif(substr($link, 0, 4)=='http') {
          //IF THE LINK STARTS WITH "https://", REMOVE IT
         if(substr($link, 0, 8)=='https://') {
              $link = substr($link, 8);
         Â
          //ELSE…IF THE LINK STARTS WITH "http://", REMOVE IT
          } elseif(substr($link, 0, 7)=='http://') {
              $link = substr($link, 7);
          }
         Â
          //ADD "/external/" TO THE BEGINNING OF THE LINK SO THAT ALL THE EXTERNAL LINKS ARE GROUPED INTO THE SAME FOLDER IN GOOGLE ANALYTICS
          $link = '/external/' . $link;
    Â
    //ELSE…IF THE LINK DOESN'T LEAD WITH A SLASH, ADD ONE (note that if the link doesn't begin with a slash, Google Analytics groups it under a folder labeled "/../")
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
}
Now we need to return the "onclick" attribute containing the tracking code for the formatted link.
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
   Â
    //RETURN THE FORMATTED LINK
    return " onclick=\"javascript:urchinTracker('$link');\"";
}
Where Does the Function Go?
Since the standard Google Analytics code is already being imported, I added the function to the "GoogleAnalytics.html" page:
//STANDARDÂ GOOGLE ANALYTICS TRACKING CODE (note that the "UA-XXXXXXX-X" needs to be replaced with your tracking code ID)
?>
<script src="http://www.google-analytics.com/urchin.js" type="text/javascript">
</script>
<script type="text/javascript">
_uacct = "UA-XXXXXXX-X";
urchinTracker();
</script>
<?php
//FUNCTION USED TO ADD TRACKING CODE TO DOWNLOADABLE FILES (SUCH AS PDFs) AND TO EXTERNAL WEBSITE LINKS
function addTrackingCode($link) {
    //IF THE LINK LEADS TO MY WEBSITE (http://www.mywebsite.com/newsletter/2010spr.pdf), REMOVE THE "http://" AND THE DOMAIN NAME
     if(substr($link, 0, 24)=='http://www.mywebsite.com') {
         $link = substr($link, 24);
         Â
    //ELSE…IF THE LINK STARTS WITH "http", IT'S AN EXTERNAL LINK
    } elseif(substr($link, 0, 4)=='http') {
          //IF THE LINK STARTS WITH "https://", REMOVE IT
         if(substr($link, 0, 8)=='https://') {
              $link = substr($link, 8);
         Â
          //ELSE…IF THE LINK STARTS WITH "http://", REMOVE IT
          } elseif(substr($link, 0, 7)=='http://') {
              $link = substr($link, 7);
          }
         Â
          //ADD "/external/" TO THE BEGINNING OF THE LINK SO THAT ALL THE EXTERNAL LINKS ARE GROUPED INTO THE SAME FOLDER IN GOOGLE ANALYTICS
          $link = '/external/' . $link;
    Â
    //ELSE…IF THE LINK DOESN'T LEAD WITH A SLASH, ADD ONE (note that if the link doesn't begin with a slash, Google Analytics groups it under a folder labeled "/../")
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
   Â
    //RETURN THE FORMATTED LINK
    return " onclick=\"javascript:urchinTracker('$link');\"";
}
?>
Using the Function
Now that the function is imported along with the standard Google Analytics code, it can be used when linking to files like PDFs. So instead of hard-coding the tracking code like earlier:
We can now utilize the PHP function:
Updating the Analytics Code
Now that all the Google-specific tracking code is located in the "GoogleAnalytics.html" page, we can easily modify the code as Google releases new versions. We just need to replace the standard tracking code and the return statement at the end. The code below shows the newest tracking code provided by Google:
//STANDARDÂ GOOGLE ANALYTICS TRACKING CODE (note that the "UA-XXXXXXX-X" needs to be replaced with your tracking code ID)
?>
<script type="text/javascript">
 var _gaq = _gaq || [];
 _gaq.push(['_setAccount', 'UA-XXXXXXX-X']);
 _gaq.push(['_trackPageview']);
 (function() {
   var ga = document.createElement('script'); ga.type = 'text/javascript'; ga.async = true;
   ga.src = ('https:' == document.location.protocol ? 'https://ssl' : 'http://www') + '.google-analytics.com/ga.js';
   var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(ga, s);
 })();
</script>
<?php
//FUNCTION USED TO ADD TRACKING CODE TO DOWNLOADABLE FILES (SUCH AS PDFs) AND TO EXTERNAL WEBSITE LINKS
function addTrackingCode($link) {
…
    //RETURN THE FORMATTED LINK
return " onclick=\"javascript:_gaq.push(['_trackPageview', '$link']);\"";
}
?>
Feedback
Do you use Google Analytics to track visits to external websites from your website? If so, do you do anything special to group those visits together? Also, don't hesitate to ask if you have any questions about the analytics function or if you have suggestions.
Related Resources
- PHP.net – the online PHP manual which is helpful for finding out more information on the PHP substr() function for example
- Google Analytics Help – the help section for Google Analytics which may be able to answer your questions about the service