Entries from 2010
Problems with Google Analytics Code for Tracking PDFs
After struggling for nearly a week to install the asynchronous code for Google Analytics, I thought it would be good to share what I've learned. Hopefully this will save you a few sleepless nights.
When I switched to the asynchronous tracking code, everything appeared to be working correctly. But for some reason PDF downloads and visits to external websites weren't showing up in the analytics. According to the Google help documentation (How do I track files (such as PDF, AVI, or WMV) that are downloaded from my site?), I just needed to add an "onclick" event to the downloadable file/external website link:
While searching for a solution I stumbled across a Google Chrome plug-in (Google Analytics Tracking Code Debugger) used to validate your tracking code. Using the plug-in, I discovered that the Google help documentation failed to mention one crucial piece of information; you need to define "pageTracker". After a little searching I found another page (Google Analytics Installation Guide) that talks about defining "pageTracker".
With "pageTracker" now defined, the Google Chrome plug-in said that everything was working. But for some reason the code still wasn't working. I used several computers to open PDFs and visit external links. Then I checked Google Analytics an hour or so later to see if they show up. For some reason one of the clicks went through, but that was it. After a few more days of failures and posting on the Google Analytics discussion board I finally realized that the tracking code provided by Google doesn't work.
Instead you want to call the tracking code directly:
I have no idea why the Google Chrome plug-in said everything was fine, maybe there is a bug on Google's side that prevents the analytics service from accepting results.
Related Links
- Future Proofing Your Google Analytics Code for Tracking PDFs – my previous post describing how I use the Google Analytics tracking code.
Stop Fighting with Me WordPress
Writing last week's post (Future Proofing Your Google Analytics Code for Tracking PDFs) introduced me to one of WordPress' major flaws. If you want to display code (HTML, PHP, etc.) in your post, there is a good chance that WordPress will mess it up.
For example, if there is a blank line in the code WordPress thinks you're starting a new paragraph and adds the paragraph tag once the post is saved. You may be able to avoid this by replacing the hard-return (Enter) with two soft-returns (Shift+Enter). But if you attempt to edit the post again, WordPress replaces the soft-returns with a hard-return causing the paragraph tag to come back.
The problem with the paragraph tag was that it broke my design. Looking at the screenshot below (Figure 1), all of the code is supposed to appear in a gray box.
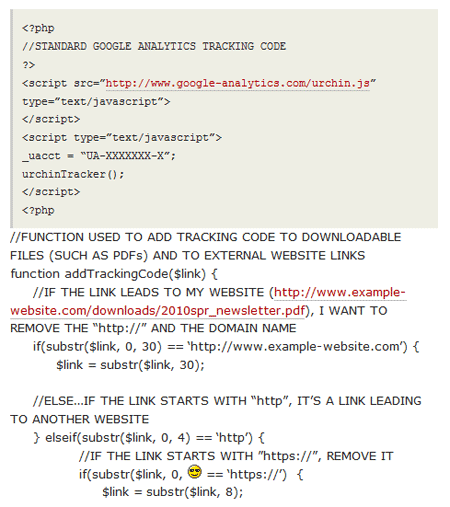
Figure 1. Broken Design Caused Paragraph Tag
In Figure 1, you may have also noticed that WordPress replaced a reference to "8)" with the corresponding Emoticon (aka the "cool" smiley face). The easy way around this issue was to avoid leaving a space after the closing parenthesis.
Preventing WordPress from Changing Your Code
Thanks to a post by Josh Stauffer titled WordPress, stop changing my HTML code!, I was able to temporarily prevent WordPress from changing aspects of my code.
Before writing (or editing) a post that displays code like HTML, you need to disable the "Visual" editor in WordPress. To disable the editor:
- Log into the admin area for your WordPress blog
- Click "Users"
- Find your profile and click "Edit"
- Check the "Disable the visual editor when writing" option (See Figure 2)
- Click the "Update Profile" button
- Write/Edit your post
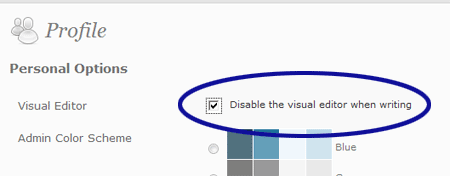
Figure 2. Disable Visual Editor Check Box
Then you just need to re-enable the visual editor for writing posts that don't need to display code. It's not an elegant solution, but it gets the job done.
Feedback
Do you have a different solution to prevent WordPress from changing code? Or maybe you want to share your WordPress struggles? If so, please enter them in the comments field below.
Future Proofing Your Google Analytics Code for Tracking PDFs
A few years back I started using Google Analytics to get a better idea of what people are viewing on our websites. To be prepared for future revisions of the tracking code, I thought all I needed to do was store the code in a file called "GoogleAnalytics.html" and import it into the pages we wanted to track. Then as Google releases new versions of the tracking code, I would only need to update the GoogleAnalytic.html file for each website.
Unfortunately, you can't add the tracking code to every file on the website. To track how many visitors open a PDF for example, you have to add extra code to the page that links to the PDF. Let's say you wanted to link to the Spring 2010 newsletter. The tracking code would likely be added to the anchor tag using the "onclick" attribute as follows:
The problem was that I didn't think about future proofing this part of the tracking code. So when the time arrived to update the analytics code, I needed to replace more than 230 onclick references throughout one website.
So instead of hard-coding the onclick attribute like before, I wanted to create a PHP function that writes the tracking code for me. In addition to being able to create tracking code for PDFs, Word documents, PowerPoint files, etc., the function should be able to handle links to other websites.
Building the Function
First I defined a PHP function called "addTrackingCode". The function accepts one argument ($link) which is used to send the link to track.
function addTrackingCode($link) {
}
Inside the function, I want optimize the link for Google Analytics.
function addTrackingCode($link) {
    //IF THE LINK LEADS TO MY WEBSITE (http://www.mywebsite.com/newsletter/2010spr.pdf), REMOVE THE "http://" AND THE DOMAIN NAME
     if(substr($link, 0, 24)=='http://www.mywebsite.com') {
         $link = substr($link, 24);
         Â
    //ELSE…IF THE LINK STARTS WITH "http", IT'S AN EXTERNAL LINK
    } elseif(substr($link, 0, 4)=='http') {
          //IF THE LINK STARTS WITH "https://", REMOVE IT
         if(substr($link, 0, 8)=='https://') {
              $link = substr($link, 8);
         Â
          //ELSE…IF THE LINK STARTS WITH "http://", REMOVE IT
          } elseif(substr($link, 0, 7)=='http://') {
              $link = substr($link, 7);
          }
         Â
          //ADD "/external/" TO THE BEGINNING OF THE LINK SO THAT ALL THE EXTERNAL LINKS ARE GROUPED INTO THE SAME FOLDER IN GOOGLE ANALYTICS
          $link = '/external/' . $link;
    Â
    //ELSE…IF THE LINK DOESN'T LEAD WITH A SLASH, ADD ONE (note that if the link doesn't begin with a slash, Google Analytics groups it under a folder labeled "/../")
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
}
Now we need to return the "onclick" attribute containing the tracking code for the formatted link.
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
   Â
    //RETURN THE FORMATTED LINK
    return " onclick=\"javascript:urchinTracker('$link');\"";
}
Where Does the Function Go?
Since the standard Google Analytics code is already being imported, I added the function to the "GoogleAnalytics.html" page:
//STANDARDÂ GOOGLE ANALYTICS TRACKING CODE (note that the "UA-XXXXXXX-X" needs to be replaced with your tracking code ID)
?>
<script src="http://www.google-analytics.com/urchin.js" type="text/javascript">
</script>
<script type="text/javascript">
_uacct = "UA-XXXXXXX-X";
urchinTracker();
</script>
<?php
//FUNCTION USED TO ADD TRACKING CODE TO DOWNLOADABLE FILES (SUCH AS PDFs) AND TO EXTERNAL WEBSITE LINKS
function addTrackingCode($link) {
    //IF THE LINK LEADS TO MY WEBSITE (http://www.mywebsite.com/newsletter/2010spr.pdf), REMOVE THE "http://" AND THE DOMAIN NAME
     if(substr($link, 0, 24)=='http://www.mywebsite.com') {
         $link = substr($link, 24);
         Â
    //ELSE…IF THE LINK STARTS WITH "http", IT'S AN EXTERNAL LINK
    } elseif(substr($link, 0, 4)=='http') {
          //IF THE LINK STARTS WITH "https://", REMOVE IT
         if(substr($link, 0, 8)=='https://') {
              $link = substr($link, 8);
         Â
          //ELSE…IF THE LINK STARTS WITH "http://", REMOVE IT
          } elseif(substr($link, 0, 7)=='http://') {
              $link = substr($link, 7);
          }
         Â
          //ADD "/external/" TO THE BEGINNING OF THE LINK SO THAT ALL THE EXTERNAL LINKS ARE GROUPED INTO THE SAME FOLDER IN GOOGLE ANALYTICS
          $link = '/external/' . $link;
    Â
    //ELSE…IF THE LINK DOESN'T LEAD WITH A SLASH, ADD ONE (note that if the link doesn't begin with a slash, Google Analytics groups it under a folder labeled "/../")
    } elseif(substr($link, 0, 1) != '/') {
         $link = '/' . $link;
    }
   Â
    //RETURN THE FORMATTED LINK
    return " onclick=\"javascript:urchinTracker('$link');\"";
}
?>
Using the Function
Now that the function is imported along with the standard Google Analytics code, it can be used when linking to files like PDFs. So instead of hard-coding the tracking code like earlier:
We can now utilize the PHP function:
Updating the Analytics Code
Now that all the Google-specific tracking code is located in the "GoogleAnalytics.html" page, we can easily modify the code as Google releases new versions. We just need to replace the standard tracking code and the return statement at the end. The code below shows the newest tracking code provided by Google:
//STANDARDÂ GOOGLE ANALYTICS TRACKING CODE (note that the "UA-XXXXXXX-X" needs to be replaced with your tracking code ID)
?>
<script type="text/javascript">
 var _gaq = _gaq || [];
 _gaq.push(['_setAccount', 'UA-XXXXXXX-X']);
 _gaq.push(['_trackPageview']);
 (function() {
   var ga = document.createElement('script'); ga.type = 'text/javascript'; ga.async = true;
   ga.src = ('https:' == document.location.protocol ? 'https://ssl' : 'http://www') + '.google-analytics.com/ga.js';
   var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(ga, s);
 })();
</script>
<?php
//FUNCTION USED TO ADD TRACKING CODE TO DOWNLOADABLE FILES (SUCH AS PDFs) AND TO EXTERNAL WEBSITE LINKS
function addTrackingCode($link) {
…
    //RETURN THE FORMATTED LINK
return " onclick=\"javascript:_gaq.push(['_trackPageview', '$link']);\"";
}
?>
Feedback
Do you use Google Analytics to track visits to external websites from your website? If so, do you do anything special to group those visits together? Also, don't hesitate to ask if you have any questions about the analytics function or if you have suggestions.
Related Resources
- PHP.net – the online PHP manual which is helpful for finding out more information on the PHP substr() function for example
- Google Analytics Help – the help section for Google Analytics which may be able to answer your questions about the service
Test Pages in the Wild
I recently listened to episode 10 of The Big Web Show titled "Less Is Always an Option". In the episode Jeffrey Zeldman and Dan Benjamin interviewed Jason Fried, CEO and co-founder of 37signals.
One thing that Jason discussed was his experience with getting feedback on web projects. He said "Until someone is really using something for real on something that needs to get done right now, they don't really put it through it's true paces. The thing that I think is missing with a lot of user testing is reality."
As I spend more time developing for the Web, I tend to agree with Jason's viewpoint. There is value in testing a website, web-based application, etc. during the development stage. For example, they may notice typos or that you don't provide a way for visitors to contact you with website questions. But the feedback quality tends to improve once the website or application is out there and being used. At this point visitors are using the product with their personal goals in mind.
If given the option, I prefer to be responsible for using the more intricate applications in the early stages. For example, I developed a tool for managing a membership database. Since I'm in charge of processing new membership requests and keeping the database updated, I've been able to experience first-hand what works well in the program and what needs to be streamlined. I can then make any necessary teaks before turning the keys over to someone else.
Feedback
How do you obtain feedback on a website or web-based application? I would also be interested in hearing your feedback stories. For example, did you launch a product only to find out that a crucial piece of information was missing?
Related Resources
- The Big Web Show: Episode 10 – the episode show notes where you can watch and/or listen to the Jason Fried interview
- Making User Testing Happen– a Boagworld post describing some of the methods being used to test websites
Creating Accessible PDFs with Word 2010
The other day I needed to post a Word document online as a PDF. After opening Microsoft Word to make the conversion I discovered that the Acrobat tab (used to create tagged PDF documents) was missing. The problem is that I’m now using Word 2010 and the process for making a tagged PDF has changed.
What is a Tagged PDF?
When you’re tagging a PDF, you’re identifying what each thing is in the document. For example, you define what elements are headers, paragraphs, graphics, etc. In the end, you should have a PDF document that is more accessible to those viewing the file with an assistive technology device such as a screen reader. As a bonus, those tagged PDF documents are also more accessible to search engines (Google, Yahoo, etc.).
Creating a Tagged PDF in Word 2010
To convert a Word document into a tagged PDF, follow these steps:
- Open the document in Word 2010
- Click File
- Click Save As
- In the Save As dialog box, change the "Save as type" to PDF (See Figure 1)
- Click Options… (See Figure 2)
- Make sure the “Document structure tags for accessibility†option is checked (See Figure 3)
- Click OK and finish saving the document
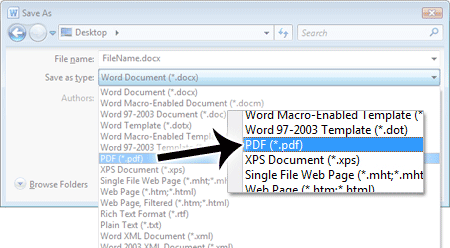
Figure 1. Save As Type Selection
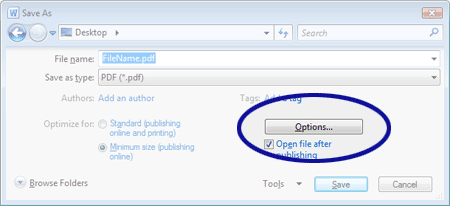
Figure 2. Save As Options Button
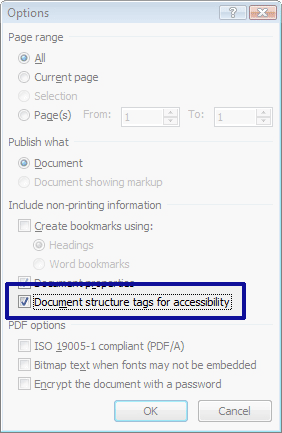
Figure 3. PDF Options Dialog Box
Once Microsoft Word has finished processing the document and added the tags, you should open the PDF file in Adobe Acrobat to verify that everything was tagged properly. Depending on how well the Word document was formatted and a number of other factors (such as document length), the verification process may take some time. The verification process is a little beyond the scope of this post, but I may post something on the topic in the future.
Related Resources
- PDF Accessibility – a WebAIM article providing an overview of the tools available in Adobe Acrobat to increase the accessibility of a PDF
Don’t Forget the Details
I recently heard an advertisment which said "Midnight movies are back starting this Friday with Indiana Jones and the Raiders of the Lost Ark." When I visited the theatre's website for the complete list of movies, I found out that Indiana Jones was shown a few weeks earlier. But there was some doubt in my mind since the website didn't list the year (See Figure 1); maybe I was looking at old information. However my confusion was cleared up when I heard the exact same advertisment over the next few weeks.
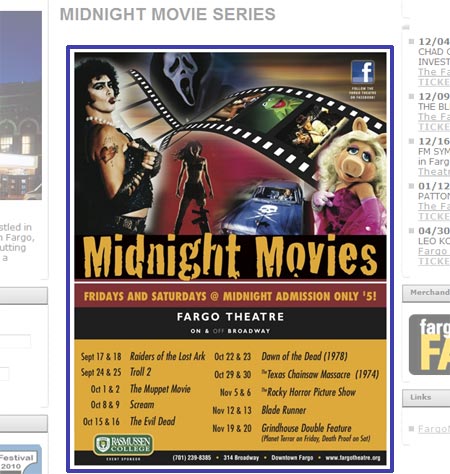
Figure 1. Midnight Movies Poster
When posting information on a website, developing a radio advertisement, etc.; it's important to step back and think like your audience. Keep in mind that they will likely to be less familiar with your organization, website, event, etc. If you were in their shoes, what questions would you need answered. Of course, you'll want to keep the message concise. If you feel like you might overwhelm your customers with too many details, you can always provide a method for them to ask questions.
So how could the message from the radio advertisement have been improved. First off, they could have mentioned the start date; "Midnight movies are back starting Friday, Sept. 17 with Indiana Jones and the Raiders of the Lost Ark." I would also recommend adding the year to the poster advertising the movie lineup.
Feedback
Do you have any examples where you felt a website, advertisement, etc. was missing critical details? Or maybe you would like to share a situation where you were in charge of something and didn't provide the necessary details; what was the outcome?
Don't Forget the Details
I recently heard an advertisment which said "Midnight movies are back starting this Friday with Indiana Jones and the Raiders of the Lost Ark." When I visited the theatre's website for the complete list of movies, I found out that Indiana Jones was shown a few weeks earlier. But there was some doubt in my mind since the website didn't list the year (See Figure 1); maybe I was looking at old information. However my confusion was cleared up when I heard the exact same advertisment over the next few weeks.
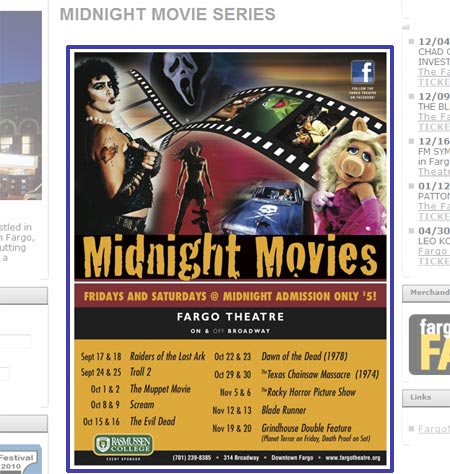
Figure 1. Midnight Movies Poster
When posting information on a website, developing a radio advertisement, etc.; it's important to step back and think like your audience. Keep in mind that they will likely to be less familiar with your organization, website, event, etc. If you were in their shoes, what questions would you need answered. Of course, you'll want to keep the message concise. If you feel like you might overwhelm your customers with too many details, you can always provide a method for them to ask questions.
So how could the message from the radio advertisement have been improved. First off, they could have mentioned the start date; "Midnight movies are back starting Friday, Sept. 17 with Indiana Jones and the Raiders of the Lost Ark." I would also recommend adding the year to the poster advertising the movie lineup.
Feedback
Do you have any examples where you felt a website, advertisement, etc. was missing critical details? Or maybe you would like to share a situation where you were in charge of something and didn't provide the necessary details; what was the outcome?
Making Meetings Work
I recently attended an online seminar called "Making Meetings Work". The following points resonated the most with me:
- Provide an Agenda – Sending a simple bulleted list of what you're going to talk about gives the meeting participants a chance to think about the topic(s) before entering the discussion.
- Agree on Action Items – As action items are identified throughout the meeting it's important to discuss the details. What needs to be done, who is responsible, when does it need to be done, etc.
- Stay on Topic– Every meeting has a time limit, so it is important to follow the agenda. If you're in charge of the meeting, you'll want to be on the lookout for unrelated tangents. You'll also want to be careful of discussions that go too in-depth. You may only have an hour to discuss several projects. If you spend a half-hour on one project, you may not have time to get to everything on the agenda.
- Create Meeting Notes – Providing meeting notes can be helpful to make sure everyone is on the same page. These notes could be as simple as a bulleted list of action items; or as in-depth as you want them to be. The notes may also provide a good starting point for the next meeting.
Meetings have become an intrinsic part of my career over the last few years. Since starting my employment with the Upper Great Plains Transportation Institute back in 2002, we have added two members to the web team. We have also established a communications group to work towards a more unified branding of our websites, newsletters, publications, etc. I don't see the need for meetings going away any time soon. So all we can do is work towards improving the efficiency of those meetings.
But is an Eraser
As a side note, the presenter talked about how to handle discussions that go beyond the scope of the meeting. She mentioned that she would say something like "You bring up a very interesting point, however it doesn't fit in with the agenda." She then discussed how she prefers the word "however" over "but". An instructor once told her that "but" is an eraser; it can seem like the point isn't as important. ("Your point may be important to you, but we don't want to hear it.")
Feedback
What techniques do you use to increase the success rate of your meetings? I would also love to hear your meeting horror stories.
Sorting Skills in LinkedIn
As mentioned in my previous post (Diving into LinkedIn), we now have the ability to add new sections to our LinkedIn profile. For a list of new sections, click the "Add Sections" link below the light blue box containing your profile picture, number of connections, public profile link, etc. (See Figure 1)
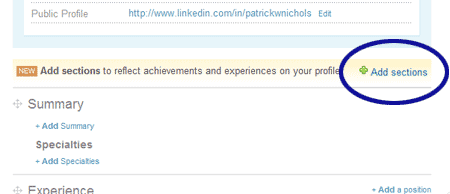
Figure 1. Add Sections Link
The most useful addition for me is the Skills section. Before LinkedIn added this section I considered listing my skill information under Specialties. Of course this wasn't the best fit since I don't specialize in some of the skills I want to list. For example, I want to list my JavaScript experience even though I don't use it very often. The new Skills section works well in this regard. (See Figure 2)
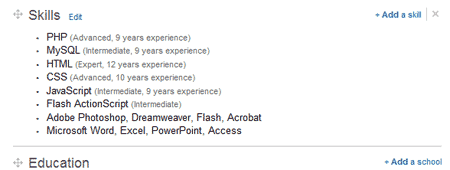
Figure 2. New Skills Section
I have one minor gripe with the Skills section though. While posting my skill information, there were several times I needed to rearrange the list. Unfortunatally, with the way things are set up I needed to move everything around manually. Note that there is a text box and two drop-down menus for each skill, so moving things around requires a little work. (See Figure 3)
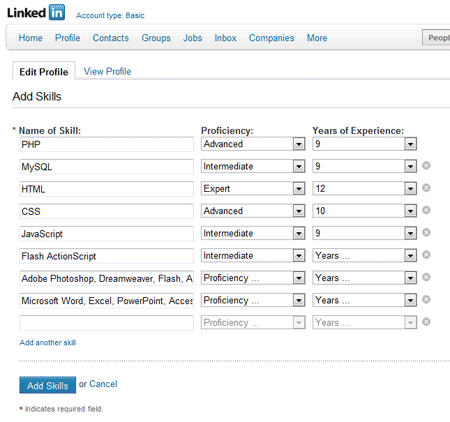
Figure 3. Edit Form for the Skills Section
There are several ways LinkedIn could improve the form to allows us to sort the list of skills. For example, Netflix added an extra input field next to each movie in the queue so you can change the list order. Or maybe LinkedIn could utilize the technique they currently use for rearranging the major sections of the profile page. To the left of the section headers (Summary, Experience, Education, etc.) there is a grey icon that can be used to drag the sections around. (See Figure 4)
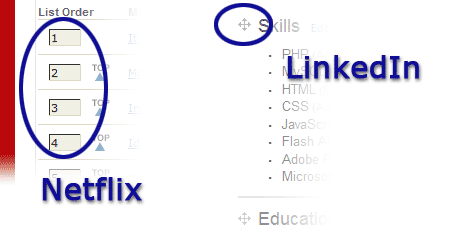
Figure 4. Netflix and LinkedIn Examples for Reorganizing Content
Feedback
Are you using the new LinkedIn sections? If so, which one do you find the most useful?
Facebook’s New Spam Filter
If you have a Community or Official Facebook Page, you've probably seen a message that says "Check Your Spam Filter – Posts likely to be spam appear here. You can review, remove or approve these posts at any time. Learn more." (See Figure 1)
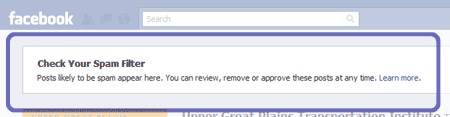
Figure 1. Spam Filter Notice
Unfortunatally, the meaning behind the message isn't very clear. My first thought upon reading the message was that someone attempted to post "spam" on my page. Then my next thought was "Facebook has a spam filter?" So naturally I started looking for the filter, but couldn't find it anywhere. When I clicked the "Learn more" link in the message, it just brought me to a Facebook help page that listed a bunch of unrelated help information. Toward the end of the list I found the information related to the spam filter, but it didn't say anything about where to find it. So the next step was to check Google.
Well I quickly found a website that mentioned the spam filter should be located under "What's on your mind?" box. I also found out that the message wasn't telling me that I had spam; instead this was Facebook's way of announcing their new spam filter.
Where is the Spam Filter?
With the way Community and Official Pages are currently set up on Facebook there is a good chance that the spam filter is hidden. Under the "What's on your mind?" box, you should see an "Options" link. (See Figure 2) If you click the "Options" link you should see the spam filter along with a few other ways to see your Wall. (See Figure 3)
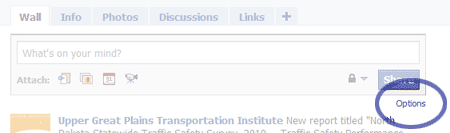
Figure 2. Options Link
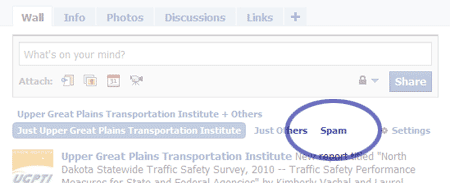
Figure 3. Spam Filter